前提
在写这篇文章前一直在思考,我对建造者模式有了一个大体的理解。但是,有没有可能会造成过度封装呢,这里还需要各位看官老爷来评判,如果想看之前的对Toast工具了的封装可以移步Android 自定义Toast,并且勘误Android工具类里面的ToastUtils,有不足之处还望指出。
话不多说先上图
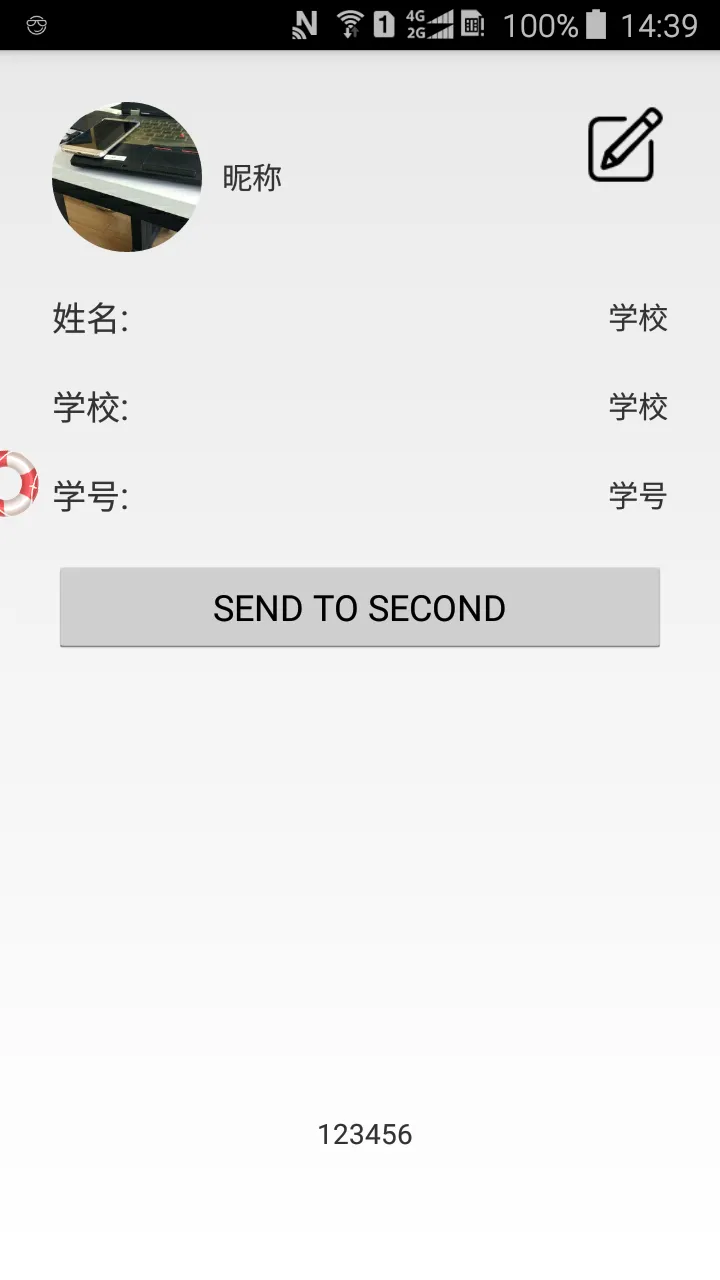


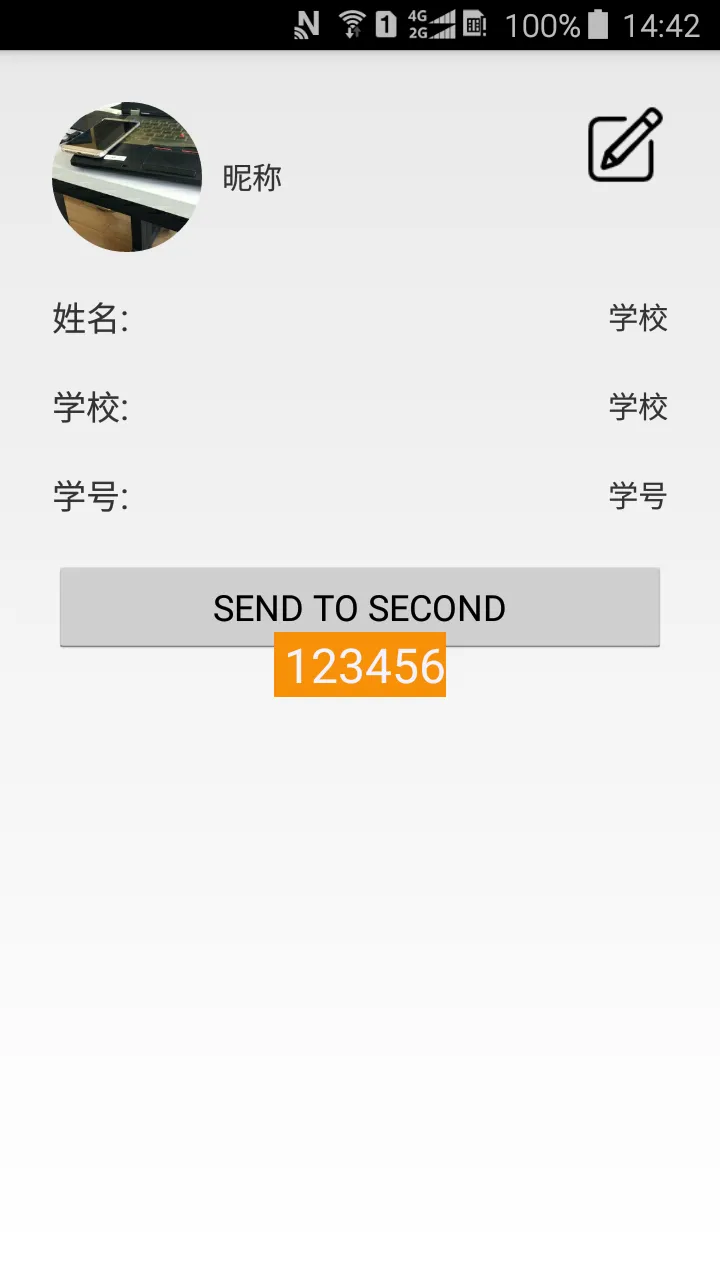
1、先讲一下什么是建造者模式
释义
建造者模式 (BuilderPattern) 又称为生成器模式,该模式主要用于将一个复杂对象的构建与它的表示分离,向用户屏蔽复杂对象组成部分的创建细节,使得同样的构建过程可以创建不同的表示。建造者模式通常包含如下4个角色。
UML图:
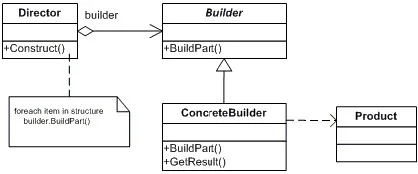
角色介绍
1.Builder:抽象建造者角色,主要为创建产品对象的各组成部分指定抽象接口,一般包含两类方法,其中 buildPartX() 用于创建复杂对象的各部分,此种方法的数量取决于复杂对象组成部分的多少;getResult() 用于返回复杂对象。
2.ConcreteBuilder:具体建造者角色,继承自抽象建造者,实现复杂对象各部件的构造和装配,并返回该对象。
3.Director:指挥者角色,客户端通常只与该角色交互,通过construct()方法方法得到复杂对象。
4.Product:产品角色(复杂对象),通常定义为一个 POJO,针对其中的每个成员对象都有一组公有的 get() 和 set() 方法。
建造者模式的分类
根据产品创建过程中零件的构造是否具有一致的先后顺序,可以将其分为“有设计者” 和 “无设计者”,两种形式。
有设计者
在现实生活中,建造一个房子,但我们不知道怎么造,就要请负责总体设计的设计师和负责具体施工的工人,设计师只设计图纸、命令工人干活,不参与施工。工人负责具体细节(窗户、地板的构建)。最后,我们要从工人手中接过建造好的房子。
对建造者(工人)的规范:
package cn.house;
public interface Builder {
/**
* 建造窗户
*/
public void mkWindow();
/**
* 建造房屋
*/
public void mkFloor();
/**
* 获取房间
*/
public Room getRoom();
}
实现了 Builder 接口的工人:
package cn.house;
public class RoomBuilder implements Builder{
private Room room = new Room();
/** 具体创建窗户 */
public void mkWindow() {
Window window = new Window();
room.setWindow(window);
}
/** 具体创建地板 */
public void mkFloor() {
Floor floor = new Floor();
room.setFloor(floor);
}
/** 交付以创建好的房子 */
public Room getRoom() {
return room;
}
}
设计师:
package cn.house;
public class Designer {
/**
* 命令 Builder
*
* @param builder
*/
public void command(Builder builder) {
// 建造房屋
builder.mkWindow();
// 建造地板
builder.mkFloor();
}
}
测试用例:
public static void main(String[] args) {
Builder builder = new RoomBuilder();
Designer design = new Designer();
design.command(builder);
Room room = builder.getRoom();
Window window = room.getWindow();
Floor floor = room.getFloor();
System.out.println(window);
System.out.println(floor);
}
无设计者
Android 中的 AlertDialog 就属于无设计者的形式,下面是 AlertDialog 的简单模拟:
public class AlertDialog {
private String title;
private String message;
private int buttonCount;
private AlertDialog() {
// empty
}
/** 获取标题 */
public String getTitle() {
return title;
}
/** 获取信息 */
public String getMessage() {
return message;
}
/** 获取按钮数 */
public int getButtonCount() {
return buttonCount;
}
/** 显示 */
public void show() {
System.out.println("show");
}
/** 建造者 */
public static class Builder {
private AlertDialog entity = new AlertDialog();
public Builder(boolean isContext) {
if (!isContext) {
throw new RuntimeException("必须有上下文");
}
}
/** 设置标题 */
public Builder setTitle(String title) {
entity.title = title;
return this;
}
/** 设置内容 */
public Builder setMessage(String message) {
entity.message = message;
return this;
}
/** 设置按钮数 */
public Builder setButtonCount(int buttonCount) {
entity.buttonCount = buttonCount;
return this;
}
/** 交付结果 */
public AlertDialog build() {
return entity;
}
}
}
可以看出,AlertDialog 直接命令 Builder ,并没有涉及到 Designer,所以它是无序的。
建造者模式的应用场景
相同的方法,不同的执行顺序,产生不同的执行效果
一个对象可以配置多个不同的零件,产生不同的效果
一个对象,参数方法极多,调用顺序不同则效果不同
Android 开源项目中的应用
由于建造者模式本身的优点,极大简化了对象的创建,一般被用于生成某些配置对象。可以看到下面的代码是多么的简洁清晰,一目了然。
2、讲解一下我们今天关于Toast的进一步封装
首先,看一下具体使用
最基本的用例:
new ToastUtil.Builder(this).setMessage("").build();
设置基本参数的用例:
new ToastUtil.Builder(this).setMessage("123456")
.setTextColor("#F2F2FF").setBackgroudColor(R.color.yellow)
.setTextSise(48).setIcon(R.drawable.ic_launcher)
.setImageSize(128).setGrivaty(Gravity.CENTER).build();
其次,让我们考虑一下,上面图中Toast显示的内容包括:文字内容、文字大小、文字颜色、图片内容、图片大小、还有背景颜色和显示位置等,那么就要定义这些变量,请各位看官来看代码(代码中比较有详细的解释,各位看官应该都可以看懂)。
public class ToastUtil {
// 消息内容
private String message;
// 图标
private int icon;
// 字体大小
private int textSize = 0;
// 字体颜色
private String textColor;
// 背景颜色
private int bgColor = 0;
// 上下文
private Context mContext;
// 是否显示
private boolean mShow = false;
// Toast
private Toast mToast;
// 布局
private LinearLayout mLayout;
// 位置
private int gravity = 0;
// ImageView
private ImageView mImgView;
// TextView
private TextView mTxtContent;
// 显示时长
private int duration = 0;
// X轴偏移量
private int floatX;
// Y轴偏移量
private int floatY;
// 图标大小
private int mImageSize;
//构造函数设置为私有的,不能直接New
private ToastUtil() {
}
/**
* Builder
*
* @author Silence
*
*/
public static class Builder {
ToastUtil mToastUtil = new ToastUtil();
public Builder(Context context) {
mToastUtil.mContext = context;
}
/**
* 消息内容
*
* @param message
* @return
*/
public Builder setMessage(String message) {
mToastUtil.message = message;
return this;
}
/**
* Toast显示位置
*
* @param gravity
* @return
*/
public Builder setGrivaty(int gravity) {
mToastUtil.gravity = gravity;
return this;
}
/**
* 显示的图标
*
* @param icon
* @return
*/
public Builder setIcon(int icon) {
mToastUtil.icon = icon;
return this;
}
/**
* 现实时长
*
* @param duration
* @return
*/
public Builder setDuration(int duration) {
mToastUtil.duration = duration;
return this;
}
/**
* 显示的字体颜色
*
* @param textColor
* @return
*/
public Builder setTextColor(String textColor) {
mToastUtil.textColor = textColor;
return this;
}
/**
* 显示的字体大小
*
* @param textSize
* @return
*/
public Builder setTextSise(int textSize) {
mToastUtil.textSize = textSize;
return this;
}
/**
* X轴偏移量
*
* @param floatX
* @return
*/
public Builder setFloatX(int floatX) {
mToastUtil.floatX = floatX;
return this;
}
/**
* Y轴偏移量
*
* @param floatY
* @return
*/
public Builder setFloatY(int floatY) {
mToastUtil.floatY = floatY;
return this;
}
/**
* 图标大小
*
* @param imageSize
* @return
*/
public Builder setImageSize(int imageSize) {
mToastUtil.mImageSize = imageSize;
return this;
}
/**
* 显示的背景颜色
*
* @param bgColor
* @return
*/
public Builder setBackgroudColor(int bgColor) {
mToastUtil.bgColor = bgColor;
return this;
}
/**
* 创建
*
* @return
*/
public ToastUtil build() {
mToastUtil.setLayoutView();
return mToastUtil;
}
}
public void setLayoutView() {
if (!mShow) {
mToast = new Toast(mContext);
// 图标
mImgView = new ImageView(mContext);
LinearLayout.LayoutParams lParams = new LinearLayout.LayoutParams(
mImageSize, mImageSize);
mImgView.setImageResource(icon);
lParams.gravity = Gravity.CENTER_HORIZONTAL
| Gravity.CENTER_VERTICAL;
lParams.setMargins(5, 5, 5, 5);
mImgView.setLayoutParams(lParams);
// 消息内容
mTxtContent = new TextView(mContext);
LinearLayout.LayoutParams lParams1 = new LinearLayout.LayoutParams(
LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
if (!TextUtils.isEmpty(textColor)) {
mTxtContent.setTextColor(Color.parseColor(textColor));
}
if (textSize != 0) {
mTxtContent.setTextSize(textSize);
}
mTxtContent.setLayoutParams(lParams1);
// 布局
mLayout = new LinearLayout(mContext);
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(
LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT);
mLayout.setOrientation(LinearLayout.HORIZONTAL);
mLayout.setLayoutParams(params);
mLayout.addView(mImgView);
mLayout.addView(mTxtContent);
if (bgColor != 0) {
mLayout.setBackgroundResource(bgColor);
}
if (gravity != 0) {
mToast.setGravity(gravity, floatX, floatY);
}
mToast.setView(mLayout);
if (duration != 0) {
mToast.setDuration(duration);
}
if (!TextUtils.isEmpty(message)) {
mTxtContent.setText(message);
}
mToast.show();
}
}
}
最后,再直接创建使用(使用建造者模式是new xx.Builder()使用的,不能用类名.setxx()使用,之前就是用的类名.setxx(),差点被自己蠢死(捂脸))
感谢
感谢博主cfanr的Android 设计模式-建造者模式
感谢博主博弈史密斯的建造者模式(侧重Java、Android)
最后啰嗦一句:设计模式在编程中很有用,应该认真思考可以写出很优雅的代码,我辈应该奋发图强,像大神们看齐。