新建ProductManageController
类
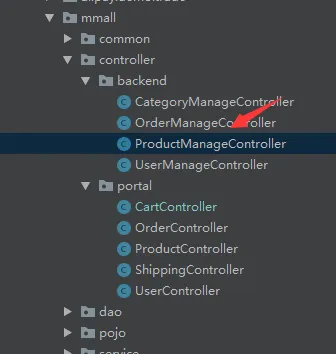
image.png
在
Controller
上添加
@Controller
和
@RequestMapping("/manage/product")
这两个注解。
@Controller
@RequestMapping("/manage/product")
public class ProductManageController {
@Autowired
private IUserService iUserService;
@Autowired
private IProductService iProductService;
@Autowired
private IFileService iFileService;
}
根据后文中要用的到Service
自动注入:
@Autowired
private IUserService iUserService;
@Autowired
private IProductService iProductService;
@Autowired
private IFileService iFileService;
1、修改或者添加商品(原来商品存在则是修改,不存在就是添加)
*Controller
:
//保存商品信息
@RequestMapping("product_save.do")
@ResponseBody
public ServerResponse productSave(HttpSession session, Product product){
User user=(User) session.getAttribute(Const.CURRENT_USER);
if(user==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),"未登录,请先登录");
}
if(iUserService.checkAdminRole(user).isSuccess()){
//增加商品的逻辑方法
System.out.println("执行。。。");
return iProductService.saveOrUpdateProduct(product);
}else {
return ServerResponse.createByErrorMessage("当前登录者不是管理员,无权限操作");
}
}
*Service
:
//修改或者添加商品方法
ServerResponse saveOrUpdateProduct(Product product);
*ServiceImpl
:
//修改或者添加商品方法
public ServerResponse saveOrUpdateProduct(Product product){
if(product!=null){
if(StringUtils.isNotBlank(product.getSubImages())){
String[] subImagesArray=product.getSubImages().split(",");
if(subImagesArray.length>0){
product.setMainImage(subImagesArray[0]);
}
}
if(product.getId()!=null){
//代表是修改商品
product.setUpdateTime(new Date());
int rowCount =productMapper.updateByPrimaryKeySelective(product);
if(rowCount>0){
return ServerResponse.createBySuccess("修改商品信息成功");
}
return ServerResponse.createBySuccess("修改商品信息失败");
}else{
// System.out.println("开始插入新增商品");
//代表是新增商品
product.setCreateTime(new Date());
product.setUpdateTime(new Date());
int rowCount=productMapper.insertSelective(product);
// System.out.println("执行完新增商品");
if(rowCount>0){
// System.out.println("插入新增商品成功");
return ServerResponse.createBySuccessMessage("新增商品成功");
}
return ServerResponse.createByErrorMessage("新增商品失败");
}
}
return ServerResponse.createByErrorMessage("新增或者更新产品参数不正确");
}
其中在修改商品的时候使用的是updateByPrimaryKeySelective
方法;
在添加商品的时候使用的是insertSelective
方法。这两个方法都是逆向工程生成的,故而不将代码贴出来~
2、上下架功能
*Controller
:
//更新商品的在售状态
@RequestMapping("set_sale_status.do")
@ResponseBody
public ServerResponse setSaleStatus(HttpSession session, Integer productId,Integer status){
User user=(User) session.getAttribute(Const.CURRENT_USER);
if(user==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),"未登录,请先登录");
}
if(iUserService.checkAdminRole(user).isSuccess()){
//增加商品的逻辑方法
return iProductService.setSaleStatus(productId,status);
}else {
return ServerResponse.createByErrorMessage("当前登录者不是管理员,无权限操作");
}
}
*Service
:
//更新商品的在售状态
ServerResponse<String> setSaleStatus(Integer productId,Integer status);
*ServiceImpl
:
//更新商品的在售状态
public ServerResponse<String> setSaleStatus(Integer productId,Integer status){
if(productId==null||status==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.ILLEGAL_ARGUMENT.getCode(),ResponseCode.ILLEGAL_ARGUMENT.getDesc());
}
Product product=new Product();
product.setId(productId);
product.setStatus(status);
int rowCount=productMapper.updateByPrimaryKeySelective(product);
if(rowCount>0){
return ServerResponse.createBySuccessMessage("修改商品销售状态成功");
}
return ServerResponse.createByErrorMessage("修改商品销售状态失败");
}
updateByPrimaryKeySelective
方法也是使用逆向工程生成的方法,故而不作多展示~
3、接口测试
1、添加商品
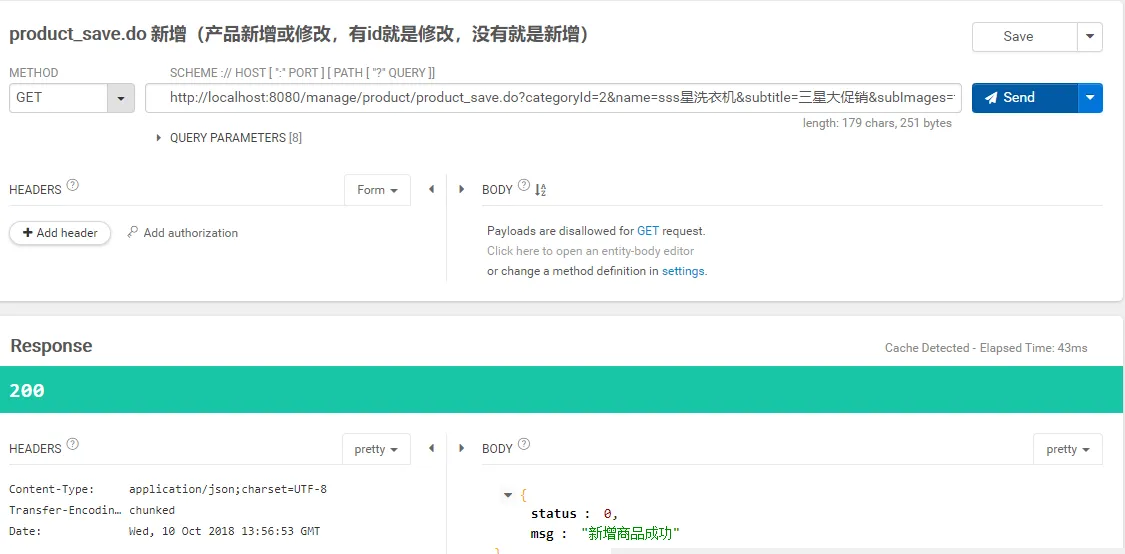
image.png
2、修改商品信息

image.png
3、产品下架

image.png
4、产品上架

image.png